I am unabashedly a couch potato. I order groceries, get food delivered, map out how long the drive is to Barnes & Noble, and check the weather to confirm that staying inside is the best course of action—all from my cozy corner of the couch. You may think that's sad, but I'd argue it's simply convenient—and I owe those conveniences to the power of APIs.
APIs, or application programming interfaces, make the conveniences of our everyday lives possible. They connect different applications, allowing them to communicate and share information effortlessly. APIs also perform a lot of the heavy lifting for programming new apps.
I worked with an expert on APIs to put together this beginner's guide and tutorial on how to use them.
Table of contents:
What is an API?
An API is like a digital mediator that enables different software applications to talk to each other and exchange information. It defines the methods, parameters, and data formats you can use to access certain features or retrieve data from an application or service.
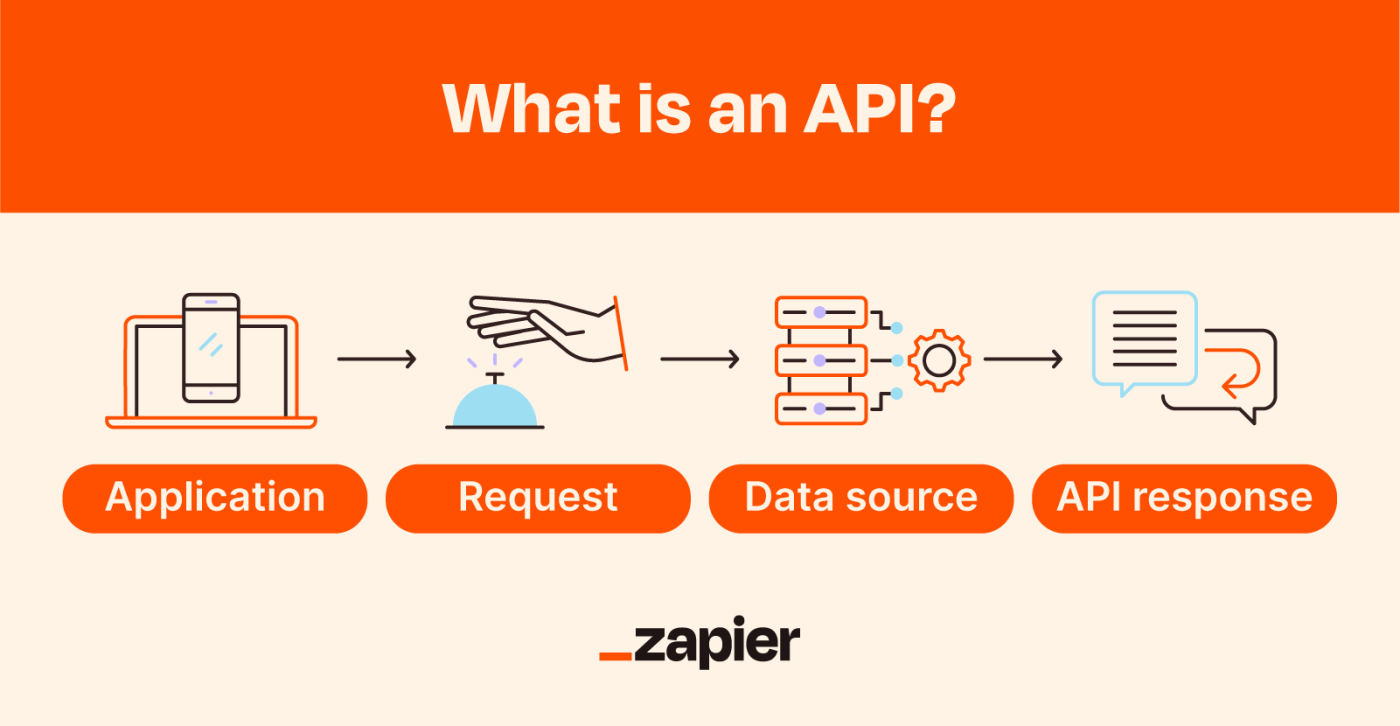
Think about your favorite weather app. It relies on APIs to fetch real-time weather data from meteorological services and deliver it to your device. Or when you use a social media platform to log in to another app (Facebook login for Candy Crush ringing any bells?), there are APIs behind the scenes working to securely authenticate your identity.
Why use an API?
Without APIs, we'd actually have to use paper maps to find our way anywhere (and I'd be lost 100% of the time). APIs let you access data from existing sources without having to reinvent the wheel. So when you use your favorite navigation app, you're actually sending a request to cell phone towers and global positioning systems (GPS) to access location data.
On the software development side, APIs offer a shortcut, allowing developers to leverage pre-built functionalities, so they can focus their efforts on building new features. For instance, Planefinder tracks planes in real time. Instead of spending their time making their own world map, they used the Google Maps API, so they could spend their time on other features.
APIs also facilitate integration between different systems, enabling seamless data exchange across apps. This comes in handy when businesses need to share information across apps—like connecting your CRM with your email. Zapier uses apps' APIs to offer thousands of no-code integrations, so all the apps in your tech stack can talk to each other (hopefully without leaving the house).
Zapier is a no-code automation tool that lets you connect your apps into automated workflows, so that every person and every business can move forward at growth speed. Learn more about how it works.
Getting started with APIs
I'll be throwing a lot of jargon your way, so before you get started, here are a few API terms you should get to know.
Term | Definition |
---|---|
API key | A unique passcode of letters and numbers that grants access to an API |
Endpoint | The digital location where an API receives requests about its resources |
Request method | Uses HTTP methods (GET, POST, PUT, DELETE) to tell the API what you want it to do |
API call | Process of a client (your computer) making an API request to a server |
Status code | A number code that appears in the body of an API response that tells you if your request was successful or not |
API keys
Like a password, an API key is a string of letters and numbers that serves as a unique access code or authentication token. You'll need one to access most APIs. It's a security measure to help track and control API usage, so only authorized users or applications can access the data. Similar to how you need a password to access your apps, you need an API key to access APIs.
We'll go over how to find and use these keys later.
Endpoints
An endpoint is where an API connects with another application, usually in the form of a specific URL or web address. Endpoints serve as the location for where requests are received and responses to those requests are sent. They're a clear and standardized way for users to work with APIs.
Imagine a vending machine. You can see all the different snacks it has inside and you have to press a specific button to receive the snack you want. An API endpoint is like a button on a vending machine, representing a particular piece of information or action you want from the API. When you "press" that button by making a request to the API endpoint, the API knows what information or action you want and provides you with the Snickers bar (er, response) you need.
Request methods
To communicate what you want to accomplish via the API endpoint, you need to make a request. There are four specific HTTP methods you can use:
GET: This is used to retrieve specific data from the API.
POST: This tells the API you want to add, or post, new data to the server.
PUT: This is used to update existing resources on the API.
DELETE: This is used to—you guessed it—delete existing data from the server.
You may also see these requests referred to as CRUD or Create, Read, Update, and Delete. Most public APIs will only let you perform GET (Read) requests to keep people from messing with their data. After all, you wouldn't want just anyone getting into a meteorology API and changing weather data.
API calls
An API call is the process of making a request, the API retrieving the data you requested, and then getting a response from the API. The only thing you have to do is make the request using one of the above HTTP methods.
Think about when you order a drink at a coffee shop. You tell the barista what you want, they put your order into the system, and then the barista hands you the drink. An API call works the same way—with a less caffeinated result.
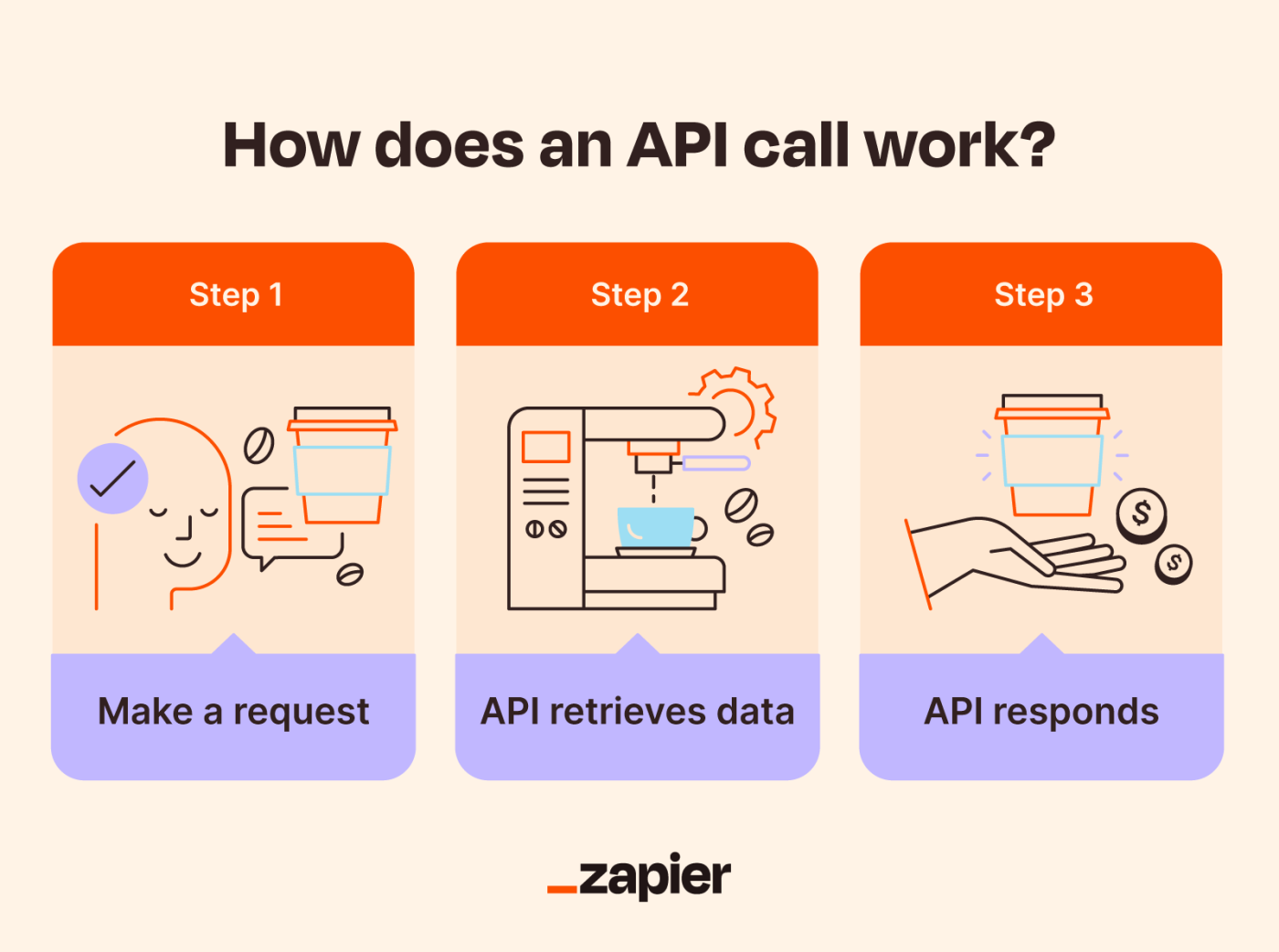
Status codes
With every request you make, you'll receive a status code. This three-digit number tells you whether or not your request was successful. The first number in the code represents the category of the status. If the code starts with a 2, your request was successfully processed. If the code starts with a 4, something went wrong. Status codes allow you to understand the outcome of your request and figure out your next move based on the response.
How to use an API
I'll be using Python (3) to show you an example of using an API. You can use other coding languages, and there are even beginner-friendly API platforms like Postman or RapidAPI (for Macs) that offer user-friendly interfaces for APIs. But Python is a pretty accessible way to use APIs, even if you're not totally fluent in the language.
1. Pick an API
There are thousands of public APIs available for use. You can search directories like GitHub or Google's API Explorer to find ones that interest you. If you're looking for a specific API, most sites will list them under Advanced settings or a Developers section, often at the bottom of the site.
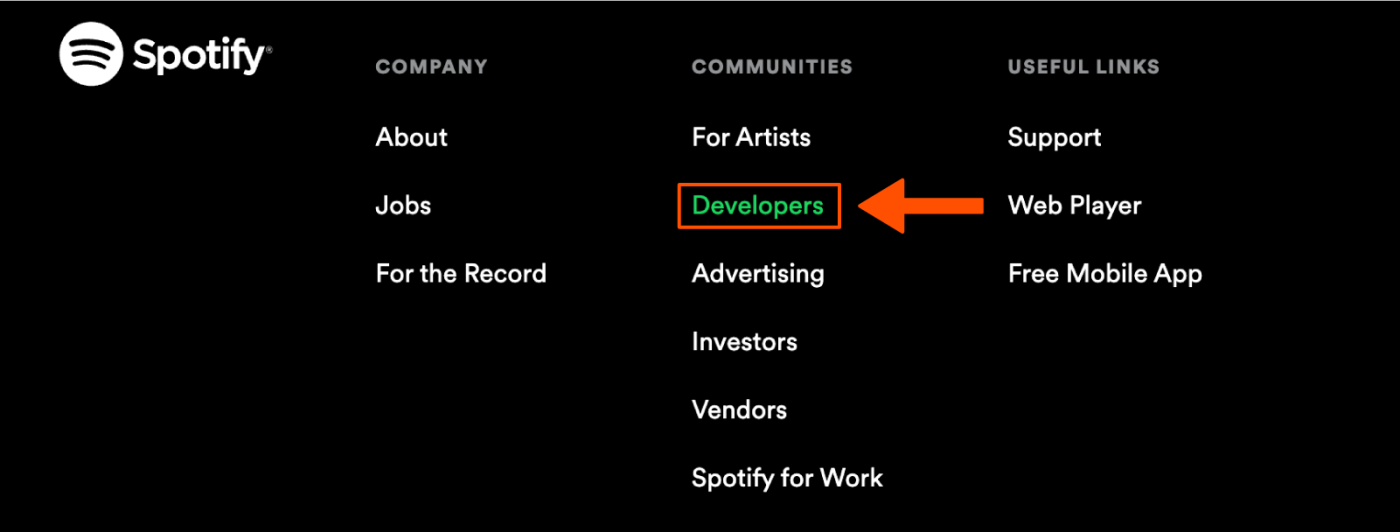
I'll be using Google's Gmail API for this tutorial.
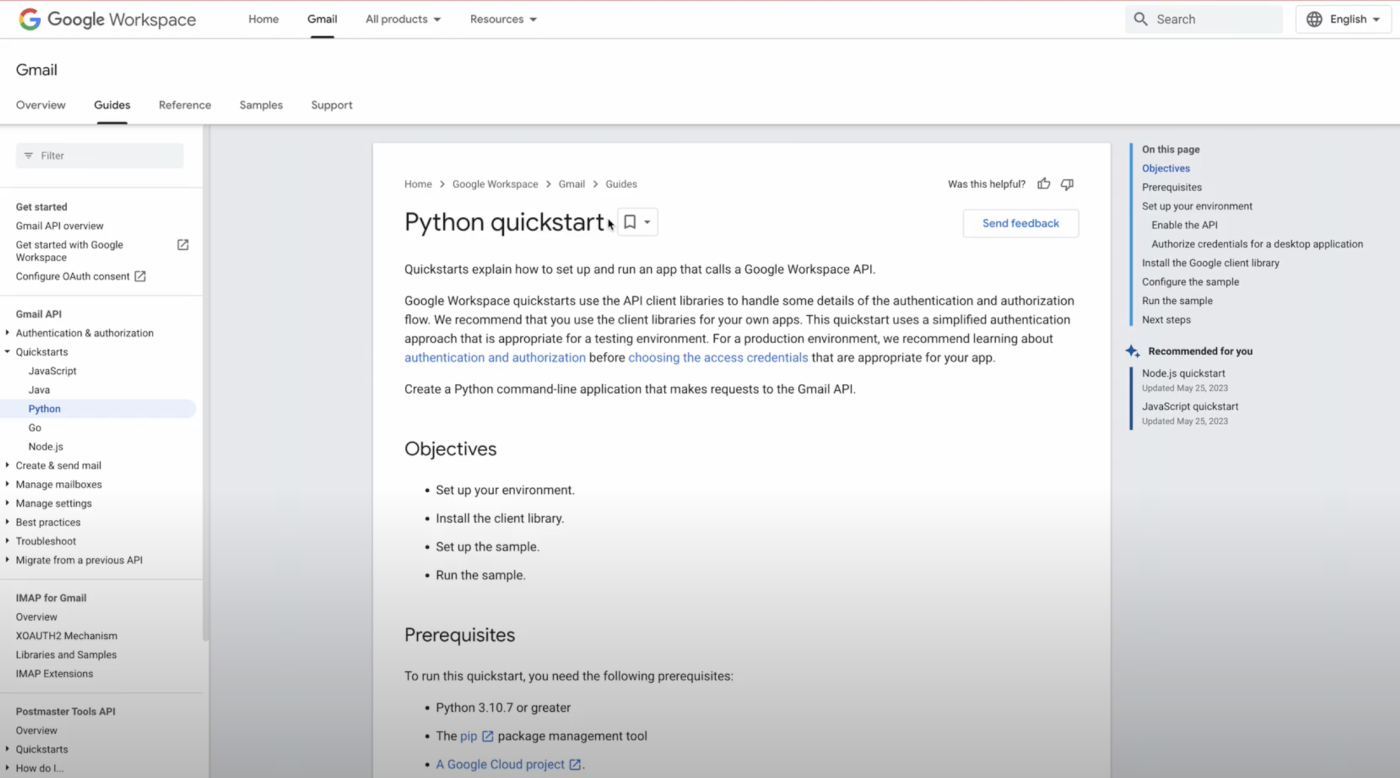
2. Consult the API documentation
Every API should provide you with some sort of documentation to start. Usually, there will be a reference section that provides the various objects, parameters, and endpoints you can access. There will also typically be an example or, in the case of the Gmail API, a "Quickstart," expressed in a few different popular languages. This document tells you:
What you can do with the API
Dependencies and requirements to use the API
How to use the API
You'll also find the API key in this document if there is one.
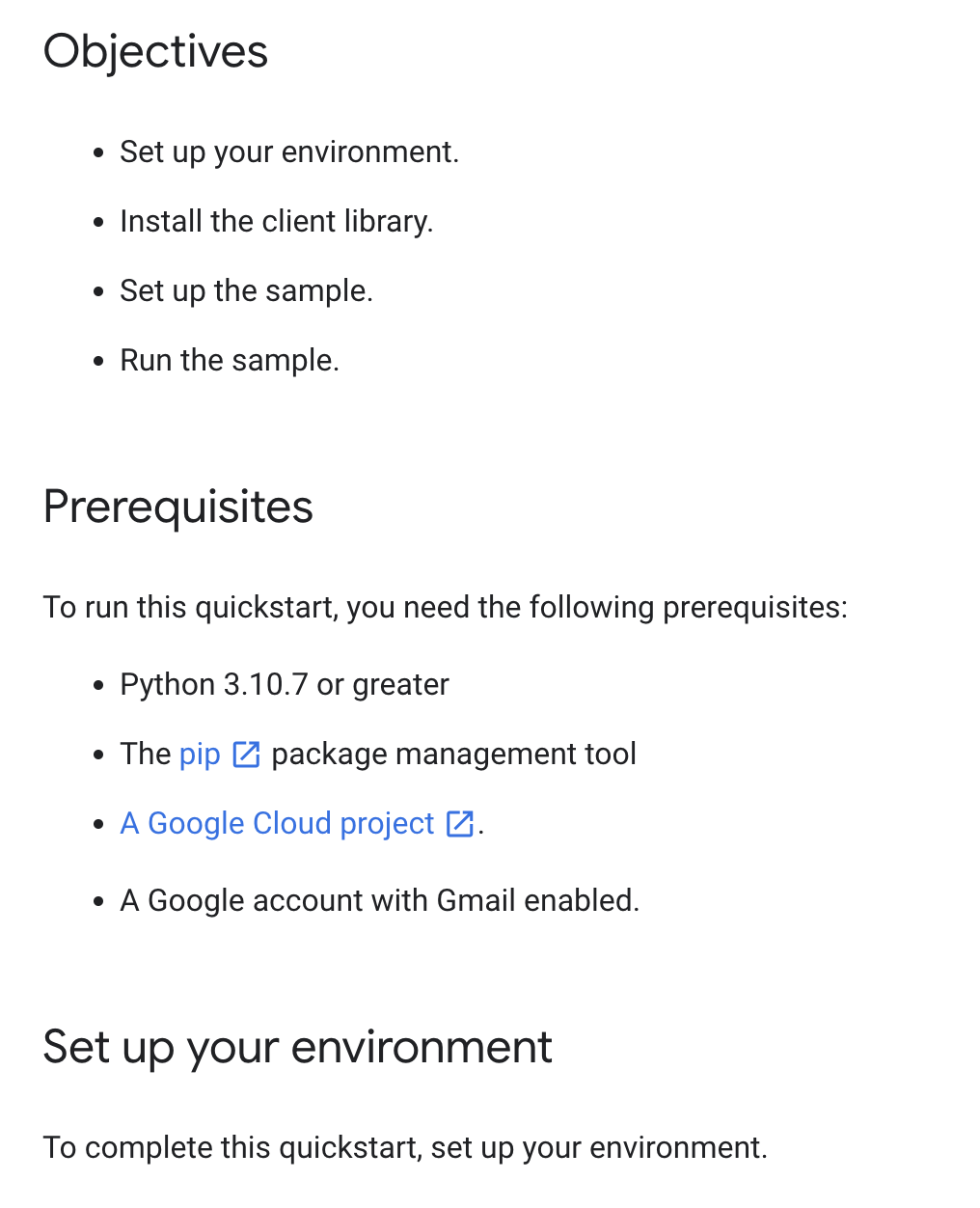
To follow along with this tutorial, you'll need to:
Install Python 3.10.7 (or a later version).
Install the pip package manager.
Create a Google Cloud project.
Create a Gmail account (if you don't already have one).
3. Write a request
Now it's time to write a request, or tell the API what you want it to do. This is where the GET, POST, PUT, and DELETE methods come into play.
Here's how to perform a GET request from Gmail's API using the boilerplate code provided by Google. This will retrieve and log the labels you have saved in Gmail to your console.
Copy the sample quickstart code.
Open a text editor like TextEdit for Mac or Notepad for Windows. Paste the code into the text editor.
Save as sample.py in the folder where you'll save all the files for this project. This is called your working directory.
Open your command line client—Terminal for Mac or Command Line for Windows—and navigate to your working directory. (Tip: In Terminal, type cd + spacebar then drag your project folder from your file system into the terminal, and press Enter.)
Type in python3 sample.py and hit Enter.
If you did everything right, it should pull up all your Gmail label data.
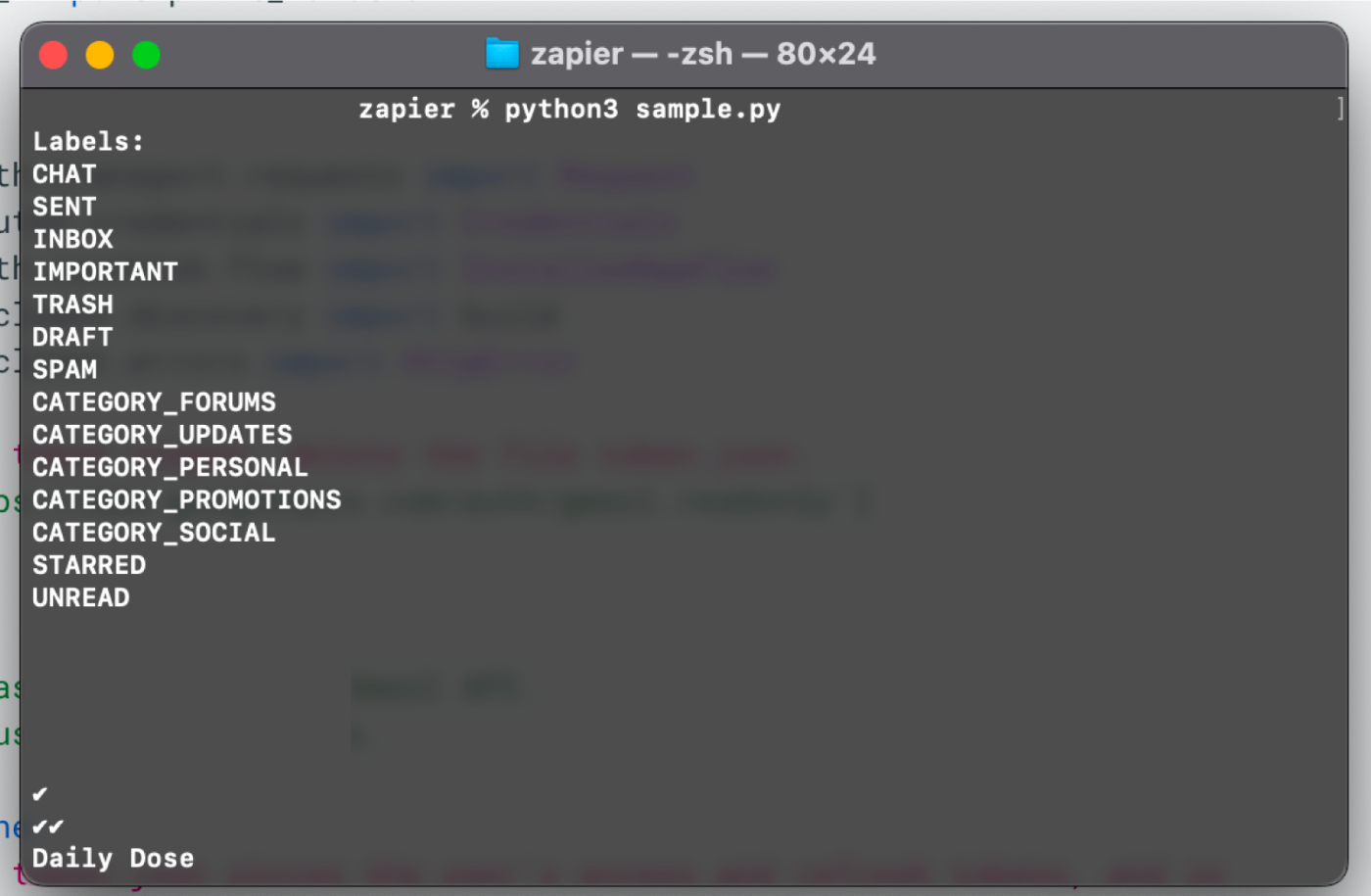
How to connect an app to an API
If you're trying to integrate applications with an API, all of the above steps will still apply. But you'll also need to:
Locate the API documentation for the apps you're trying to connect.
Find the API key (if there is one).
Follow the protocol listed (e.g., install dependencies) in the documentation.
Use a command line to run the request and return the API response.
Of course, you don't really need to go to all that trouble when you can just connect your apps using Zapier instead.
REST vs. GraphQL vs. SOAP APIs
A REST (representational state transfer) API, or RESTful API, is the most common form of API today. It's web-based, meaning the information is exchanged online. When you make a request with a REST API, you'll receive all of the data available from the API.
GraphQL (query language) APIs work in a similar fashion, except they only fetch and respond with the specific data you want instead of getting all of it. So you can specify the fields you want and avoid large data exchange and a subsequent load speed headache. GraphQL APIs are fairly new to the scene and therefore not as common, but are growing in popularity.
SOAP (simple object access protocol) APIs use XML (extensible markup language), a type of programming language, to communicate. They were the standardized approach for communicating between different software applications in the early 2000s. They've significantly declined in popularity since then, as they're more complex and inflexible compared to alternatives like REST and GraphQL.
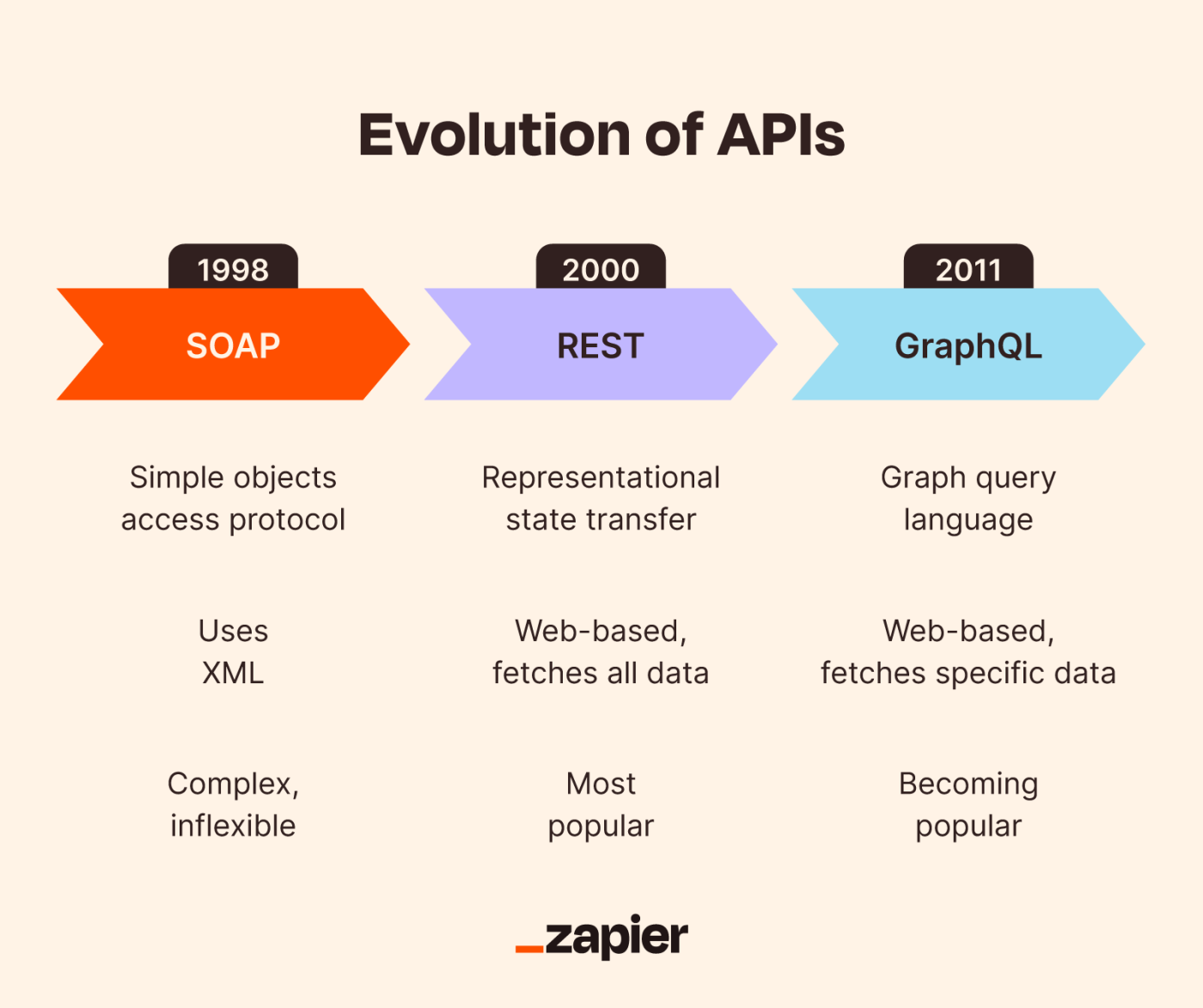
Types of APIs
There are four ways APIs are categorized, each with its own users and privileges.
Open APIs: These are public APIs that let developers access the platform's services or data. They're also accessible for third-party integrations.
Example: Google Maps API—anyone can use this API to integrate Google Maps into their apps to provide access to mapping and location-based services.
Partner APIs: This is a type of API created for partners or selected third-party entities. They provide restricted access to certain functionalities or data, often for integration between the platform and its partners.
Example: Facebook Login API—third-party applications can use it to enable user authentication with Facebook credentials for an easy login experience.
Internal APIs: These are also known as private APIs, and they're created and used by an organization for internal purposes. They facilitate communication and data sharing between internal systems.
Example: Amazon API—Amazon employees share data exclusively through their internal APIs, helping them scale over the years.
Composite APIs: Also referred to as orchestration APIs, composite APIs consolidate data or functionalities from multiple APIs. They act as a single entry point for clients and simplify the complexity of interacting with multiple APIs with a unified interface.
Example: With Twilio API, multiple communication channels are integrated, allowing users to build comprehensive communication solutions with one API.
APIs open up endless opportunities to create innovative applications and services. But if you just want to use APIs to integrate your apps or automate your workflows, you can skip the coding and use Zapier to connect any app—from the comfort of your couch.
Related articles: