I don't know about you, but the highlight of my day is completing mind-numbing and repetitive tasks—manual data entry, downloading hundreds of images one-by-one, and searching every file on my desktop for the right document.
Can't relate? Weird. But lucky for you, we live in a time where computers can help with the busywork we've become so accustomed to doing by hand. Python is a particular favorite programming language among those new to task automation.
I consulted with a Python expert to develop nine Python automation scripts, each of which can automate tasks you've been doing manually for way too long.
Related reading: Not sure when to automate a task? Start here.
Why automate tasks with Python?
Automation lets you hand off business-critical tasks to the robots, so you can focus on the most important items on your to-do list—the ones that require active thought and engagement. No-code automation should be your first stop, but Python's popularity for task automation comes from a variety of factors, including its:
Simplicity and intuitiveness: Compared to many other programming languages, Python is very easy to read and comprehend. While learning a language like C++ or Java can feel like learning a foreign language, Python syntax resembles English.
Support of data structures: Python offers several ways to store data—including lists and dictionaries—and the ability to create your own data structures. This makes data management easy, improving automation responsiveness.
Extensive automation capabilities: Python comes equipped with a huge set of libraries that enable you to accomplish nearly any automation goal that comes to mind—machine learning, operating system management, and more. Plus, Python's support network is huge, so you should be able to find an answer to nearly any automation question online.
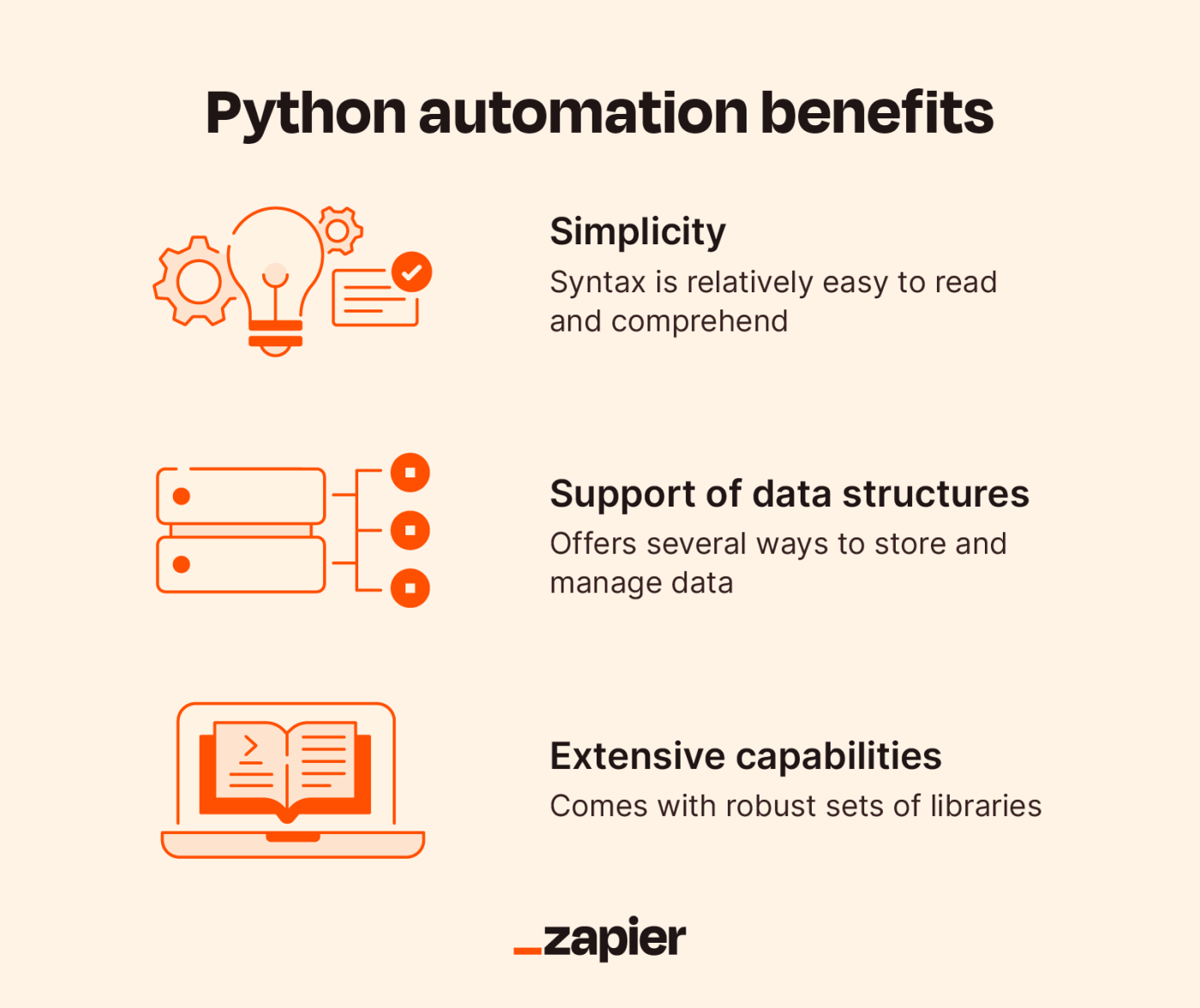
How to run a Python script
Before you start creating scripts, here's a little refresher on how to use Python. First, download Python onto your device (for free!). Once you download it, you can create and run a script.
Your script file needs to be named with the extension .py
, which stands for "Python." This tells your device that the file will contain Python code. You'll then add your script to this file and run it using your device's command-line or terminal.
After creating your file and opening your terminal, type python3
followed by the path to your script. For example, on my Mac, I would:
Create an empty text file called
FILE.py
.Add my script
Open the Terminal app
Type
python3
Drag the file into the app, and press enter, which would run the script.
I created a simple script as an example. Here's what the file looks like:
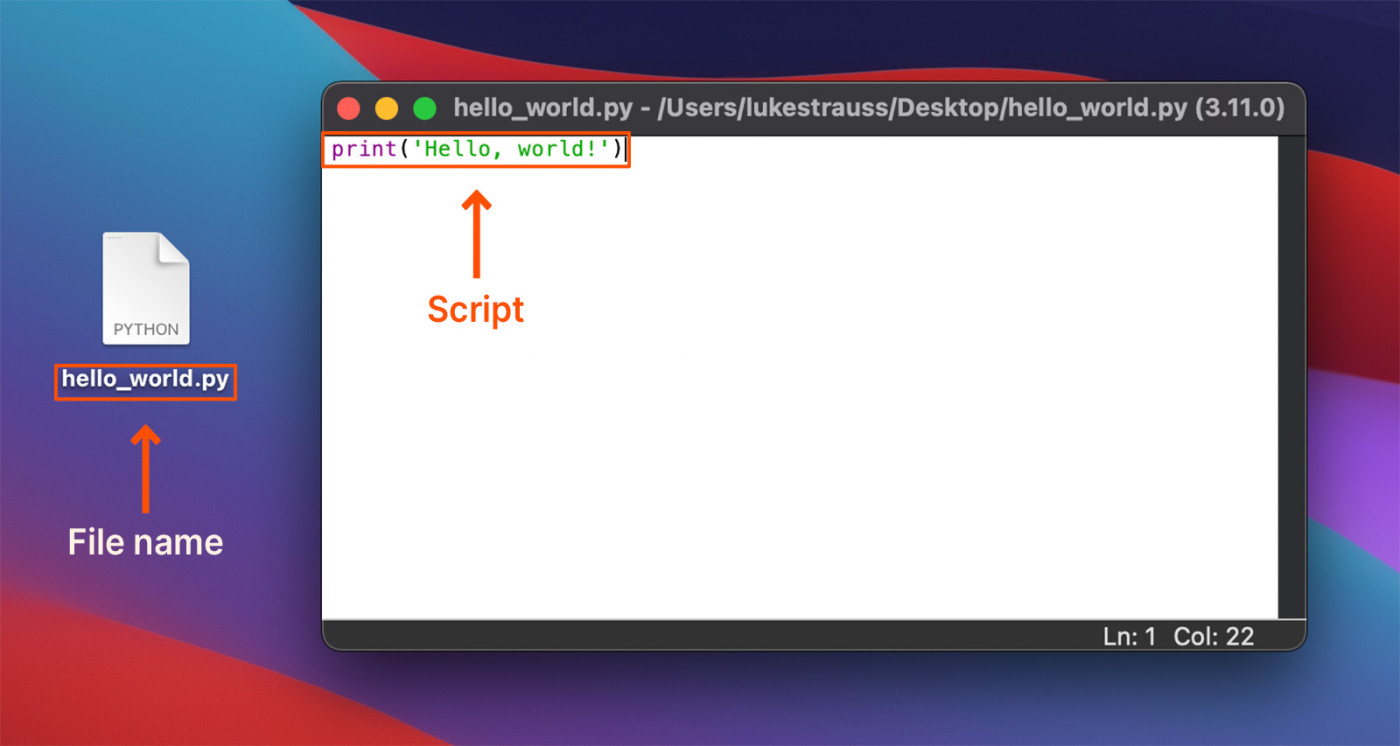
And here's what it looks like to execute the script in Terminal:

If you're running one of the most recent versions of Windows, you should be able to type your .py file's name in the Windows Command Prompt and run it without first typing the python3
command. And voilá—you're ready to get automating.
9 useful Python automation script examples
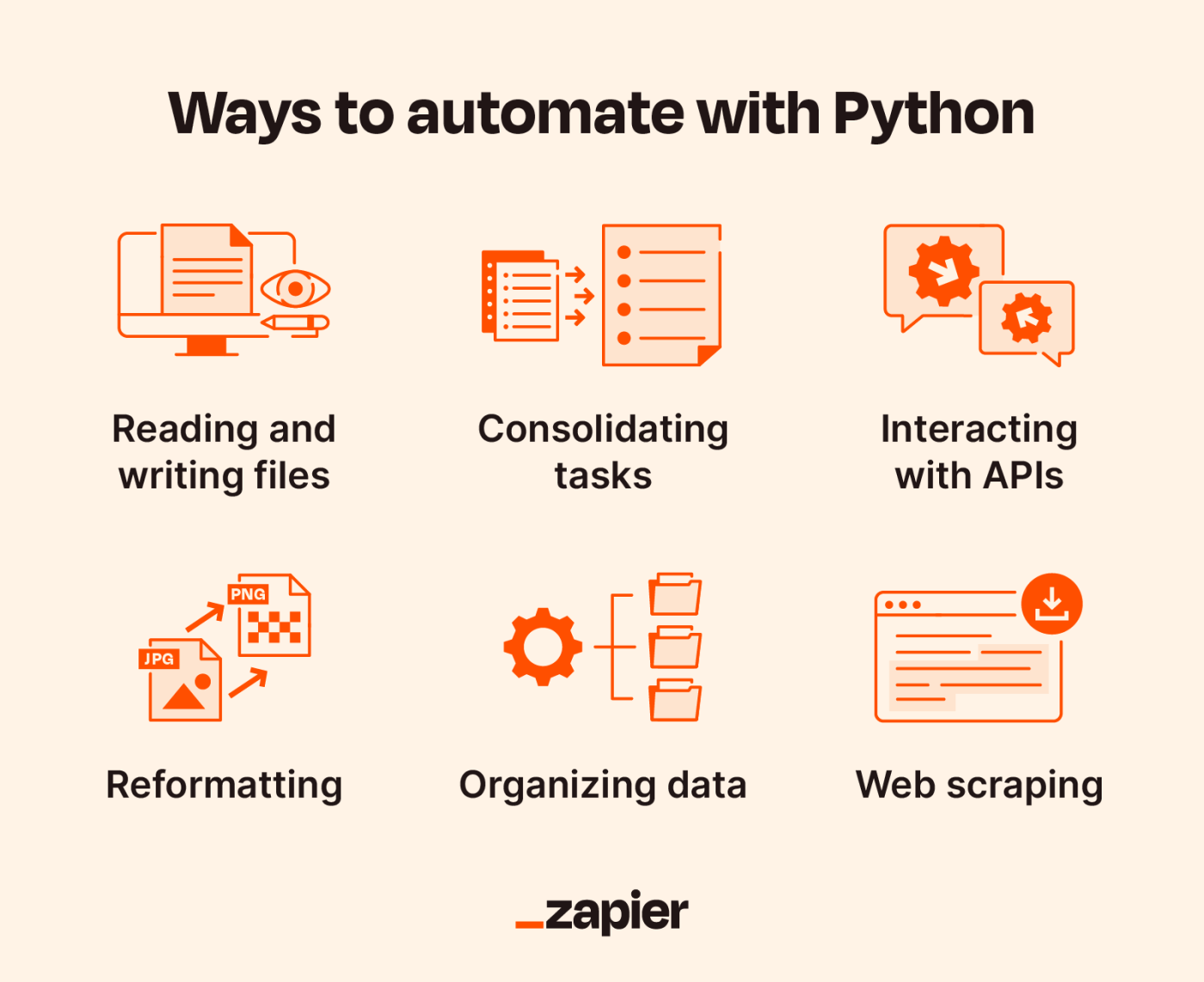
Uses of programming languages are practically unlimited, but nobody has time to learn every script there is. I've compiled nine Python automation ideas to simplify tasks that may otherwise distract you from important work, grouped into the following categories:
Interacting with APIs
Web scraping
Reformatting
Reading and writing files
Consolidating tasks
Organizing data
Running these scripts may require some preparatory steps, like downloading libraries. Follow the directions below, and you'll have Python completing tasks for you in no time.
1. Pull live traffic data
APIs make it possible to retrieve real-time data from third parties. Traffic data is always changing, so it presents a great opportunity to work with an API. With Python, we can quickly pull live traffic data as long as we have the URL where the API collects the data. For this example, I used TomTom's API URL that enables access to live Los Angeles traffic data.
In the following script, I first install the requests library in order to gather data from the URL, then unpack the URL as a JSON file.
Requests library:
|
|
Automation goal: Interacting with APIs
2. Compile data from a webpage
If your workday involves regularly pulling fresh data from the same websites, Python's web scraping capabilities can save you a lot of time. While it has specialized libraries to extract from specific sources like Wikipedia, the following script uses a more versatile web parsing and scraping library called BeautifulSoup.
Before you can extract a page's data, you first have to download it with Python's requests library. Refer to the command from the script above to download the requests library if you haven't yet, and the command below to install the BeautifulSoup library.
BeautifulSoup library:
pip or pip3 install beautifulsoup4
With all the tools we need installed, we can use the requests library to download the contents of the page of interest. Let's say you wanted to pull today's headlines from the BBC News Home page. Here's a script to download its contents.
import requests
response = requests.get('https://www.bbc.com/news')
print(response.status_code)
If Python outputs the status code 200, that means it successfully downloaded the page's contents. Theoretically, at this point, you could just type response.content
to see all of the page's contents. But, chances are, you want to see specific elements on the page rather than a big wall of HTML, which is where BeautifulSoup comes in.
Import BeautifulSoup and create an object called soup
that parses HTML pages (since BBC uses HTML) using the following commands:
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.content, 'html.parser')
print(soup)
The page is now saved in your soup
element, and you can extract whatever data you need from it. There are a lot of commands that enable you to pull specific types of data. For example, if you wanted to pull all of the H2 news headings from the site, you could input:
print(soup.find_all('h2'))
Play around with the BeautifulSoup library to pull whatever data you need from a webpage with the punch of a command.
Automation goal: Web scraping
3. Convert PDF to audio file
For the visually impaired (or for those of us who would rather listen to an audiobook than pick up a physical copy), Python offers libraries that make text-to-speech a breeze. For this script, we'll be pulling from PyPDF—a library that can read text from PDFs—and Pyttsx3, which converts text to speech.
The below script takes a PDF, removes spaces and lines that would impede Pyttsx3's ability to read the text naturally, and converts the PDF to an audio file. If you were to implement some web scraping script, you could also use PyPDF and Pyttsx3 to convert a webpage into an audio file.
|
Automation goal: Reformatting
4. Convert a JPG to a PNG
Let's say you want to improve a JPG image's capacity for high color quality and clarity by converting it to a PNG. With Python, changing the format of an image is simple. First, install the PIL package with the following command:
pip or pip3 install Pillow
Next, run the following script, filling in the placeholder path next to Image.open
with the path to the image you want to modify, and the placeholder path next to im1.save
with the path of the location you want to save your new PNG.
|
Automation goal: Reformatting
5. Read a CSV
CSV (Comma Separated Values) is a common format for importing and exporting spreadsheets from programs like Excel. Python can read a CSV, meaning that it can copy and store its contents. Once Python reads the spreadsheet's contents, you can pull from these contents and use them to complete other tasks.
|
The above script would read and print out the contents of the CSV file "customers.csv," adding commas between them.
Automation goal: Reading and writing files
6. Modify a CSV
You can also modify an existing CSV file using Python's write
feature. This replaces the contents in a CSV file without needing to enter new data manually. Learn more about how to read and write CSV files using Python's CSV library.
|
Automation goal: Reading and writing files
7. Sending personalized emails to multiple people
Nobody likes sending 30+ nearly identical emails, one-by-one. If you work in a field that requires this—marketing, education, or management, to name a few—Python makes this significantly easier. Start by creating a new CSV and filling it with all of your recipients' information, then run the script below.
You may have to create an app password for Gmail to run the script. If you notice the script doesn't run without one, create your password and enter it following the prompt Enter password:
in the script.
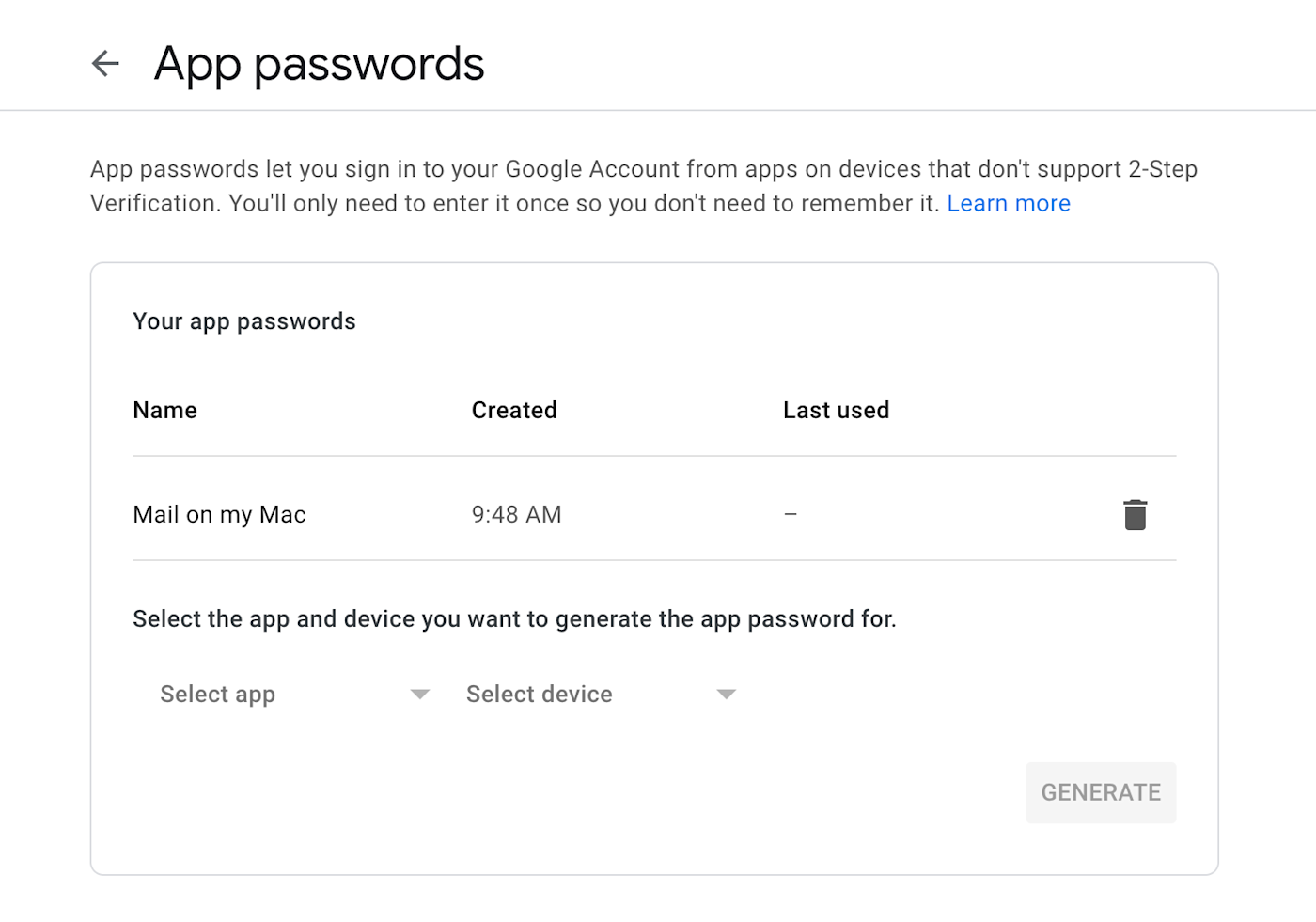
|
Automation goal: Consolidating tasks
8. Bulk uploading files to a cloud-based platform
While uploading a single file to the cloud likely isn't causing you a whole lot of grief, manually uploading tens or hundreds of files at a time can eat away at valuable time. Luckily, Python's requests library (which we've pulled from in earlier scripts) can simplify this process.
In the example below, we'll upload files to Google Drive specifically. In order for Google's authentication process to work, you'll need to set up a project and credentials in your Google Cloud Dashboard. Here's a quick overview of how to do this:
In an existing or new Google Cloud Project, navigate to Library > Search "Google Drive API," and click Enable.
Navigate to Credentials > + Create Credentials > OAuth client ID
Create a consent screen by providing the required fields.
Repeat step two, this time selecting Application Type of Desktop App, and click Create.
Download the auth JSON file, and place it in the root of the project alongside the .py script below.
pip or pip3 install pydrive
|
Automation goal: Consolidating tasks
9. Cleaning up your computer
If you're like me and your computer desktop looks like a warzone, Python can help you organize your life in a matter of seconds. We'll be using Python's OS and shutil modules for this process, as they allow Python to make changes to your device's operating system—think renaming files, creating folders, etc.—and organize files.
The below script creates a folder titled "Everything" on your desktop and moves all of the files into it. We're keeping it simple here, but feel free to play around with Python's OS and shutil modules to better organize your files.
|
Automation goal: Organizing data
Taking automation to the next level with Zapier
Zapier is equipped to automate a lot of processes—probably more than you know exist. But for those that aren't directly built into Zapier, you can use Code by Zapier to create triggers and actions using JavaScript or Python.
It's also very likely you can automate your workflows without any code at all. Zapier's no-code platform lets you automate your business-critical work with a visual builder—no code required.
Related reading: